Redux has long been a go-to framework for managing state in React applications, but anyone who’s used it knows that it can come with a lot of boilerplate. Setting up stores, creating actions, handling reducers, and managing middleware can become time-consuming and complex. Redux Toolkit (RTK) is designed to change that—it’s a popular tool that simplifies Redux development while still sticking to core Redux principles.
With RTK, developers can skip a lot of the setup work that Redux traditionally required. It brings a set of utilities that makes Redux more approachable and less error-prone, while promoting best practices. It’s essentially an all-in-one package for managing Redux with fewer headaches.
How Does Redux Toolkit Achieve This?
RTK isn’t just about reducing code—it’s about making Redux easier to use in several core areas:
- Simplified Store Configuration
One of the first pain points with Redux is setting up the store and adding middleware. With RTK’s configureStore, setting up a store is straightforward. It has built-in support for adding common middleware (like Redux Thunk) and development tools (like Redux DevTools), saving you from having to configure these yourself.
- Reducer and Action Creation with createSlice
Another feature that stands out is createSlice. Traditionally, Redux actions and reducers required a lot of boilerplate to keep things organized, but with createSlice, you can handle actions and reducers together. This means less code and a more streamlined approach to defining your application’s state.
- Intuitive State Updates Using Immer
Redux encourages immutable state, which means you generally have to create new objects each time state updates. RTK integrates Immer, a library that allows you to write mutable code that’s converted under the hood into immutable updates. It lets you write simpler code that avoids common mistakes, especially in complex updates, while still following Redux principles.
- Middleware and Selector Optimization
RTK also comes with Redux Thunk for asynchronous actions and reselect for memoized selectors by default. Selectors are crucial for performance because they help you efficiently retrieve data from the state. Memoization ensures that you’re not recalculating state unless the underlying data changes.
- Advanced Data Management with RTK Query
For applications with frequent data fetching needs, RTK Query is a game-changer. It provides a standardized way to handle data fetching, caching, and synchronization, making it much simpler to work with server state without having to set up custom logic from scratch.
What Are the Benefits of Using Redux Toolkit?
So why use RTK in your next project? Here are a few reasons:
- Convenience: RTK removes much of the boilerplate code Redux is known for, especially with createSlice.
- Performance Enhancements: By supporting memoized selectors and Immer, RTK optimizes Redux while maintaining immutability.
- User-Friendly API: The API is much cleaner, and the learning curve is gentler than vanilla Redux. Developers can focus more on the logic and less on setup.
- Large Community Support: RTK has become the standard for Redux, so there’s a large community actively supporting it, which makes finding help and resources easy.
- Comprehensive Documentation: The documentation is thorough, with clear examples and explanations, making it easier for new users to understand and implement.
Are There Drawbacks?
Of course, RTK isn’t without its limitations, and it may not be the right choice for every project.
- Larger Bundle Size: RTK adds some weight due to its abstractions (The bundle size increase when using Redux Toolkit compared to a traditional Redux application is typically around 10-20 KB), although for most modern apps, this is negligible.
- Reduced Customization: RTK’s abstractions are helpful, but for complex applications that require a high degree of customization, they might feel restrictive.
- Learning Curve: While RTK is more approachable than vanilla Redux, there’s still a learning curve, especially with RTK Query and custom middleware configurations.
- Overkill for Small Apps: If you’re working on a simple app with minimal state, Redux or RTK might not be necessary at all. For smaller state requirements, something like React’s useState and useReducer might be sufficient.
Let’s set up a Redux Toolkit Project
First start with installing the necessary dependencies.

Configuring a Redux store

The Redux store holds your application’s single source of truth, and this setup establishes the central hub for your Redux state management. Previously, createStore was used to create it, but the recommended approach now is configureStore, which simplifies store creation and accepts reducer functions.
Creating slices with reducers, actions and selectors
A slice represents a part of the global state and combines a reducer with its related actions. You can create a slice using the createSlice function.
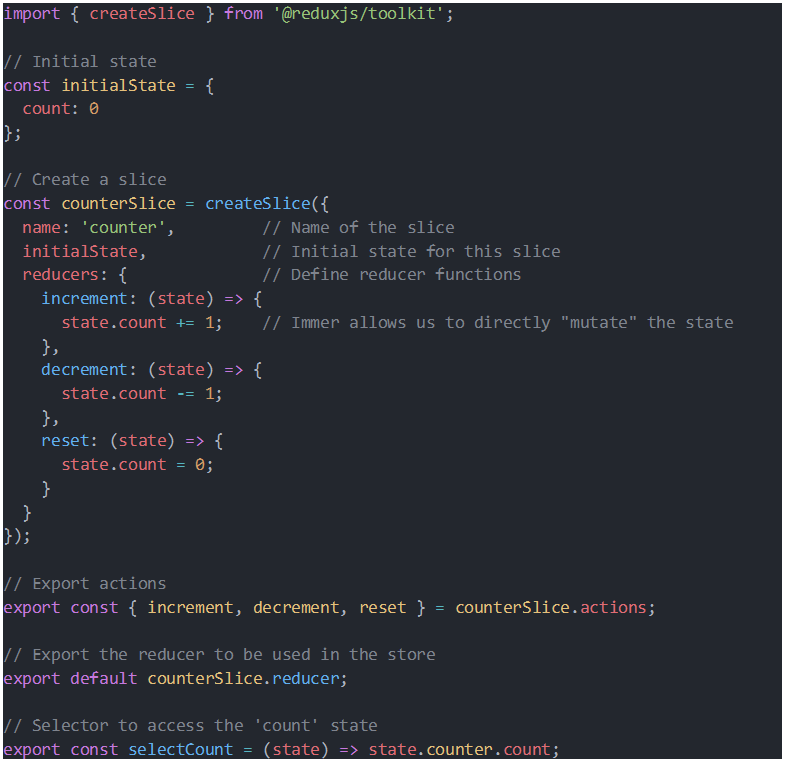
Explanation:
createSlice
is used to create the slice. It requires aname
,initialState
, andreducers
.- Reducers like
increment
,decrement
, andreset
handle specific state changes. - Actions and the reducer are automatically generated, making it easier to manage state changes.
This can be integrated into your Redux store by adding the reducer to your store configuration. And the action creators generated by createSlice
can be exported to use in other components.
Here you can also see selectors being used. Selectors in Redux are functions that allow you to extract and read specific pieces of state from the Redux store. When working with slices in Redux Toolkit, selectors make it easy to retrieve parts of the state related to a specific slice.
Instead of accessing the entire Redux state object and manually navigating through it to get specific values, selectors let you write reusable functions that return only the data you need. This keeps your code cleaner and more maintainable.
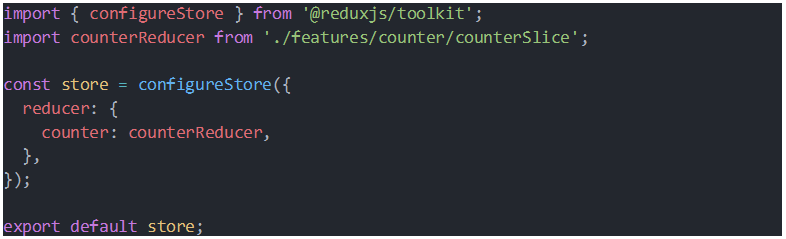
This code illustrates how to register a slice in the Redux store. By adding the counterReducer from the counterSlice to the reducer object, the store is set up to manage the counter state. This configuration allows the store to process actions related to the counterSlice.
Adding createAsyncThunk to Your Redux Slices: Simplifying Async Logic
In Redux, managing asynchronous actions like fetching API data or handling time-consuming operations used to be a bit of a chore. Typically, you’d bring in middleware like redux-thunk
, define multiple action types (loading, success, error), and then write action creators to handle the flow of these actions. It wasn’t exactly simple or intuitive, especially for developers new to Redux.
Now with Redux Toolkit’s createAsyncThunk
, async actions become far easier to manage. This function is a powerful tool that automatically creates the necessary action types and handles dispatching actions for each stage of the async request lifecycle—pending, fulfilled, and rejected. In essence, createAsyncThunk
abstracts away most of the boilerplate, letting you focus on what the action should do rather than how to orchestrate it.
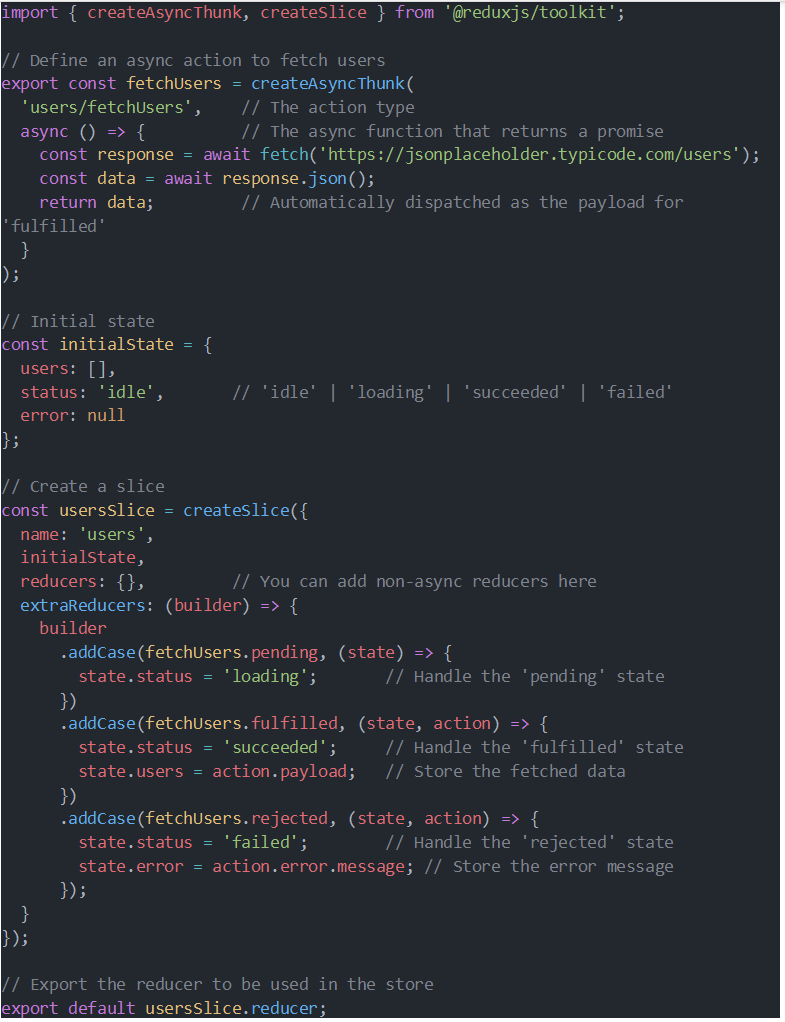
The fetchUsers thunk manages asynchronous API requests by generating action types for the pending, fulfilled, and rejected states. The usersSlice utilizes these states to appropriately update its status, users, and error fields. This approach streamlines asynchronous operations in Redux.
Now add the user reducer to the store.
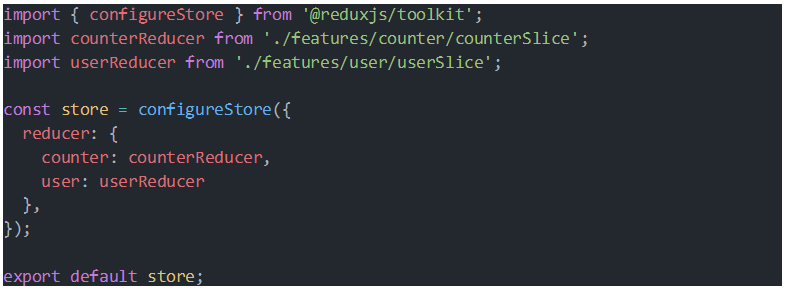
Interacting with the Redux Store Using useSelector and useDispatch
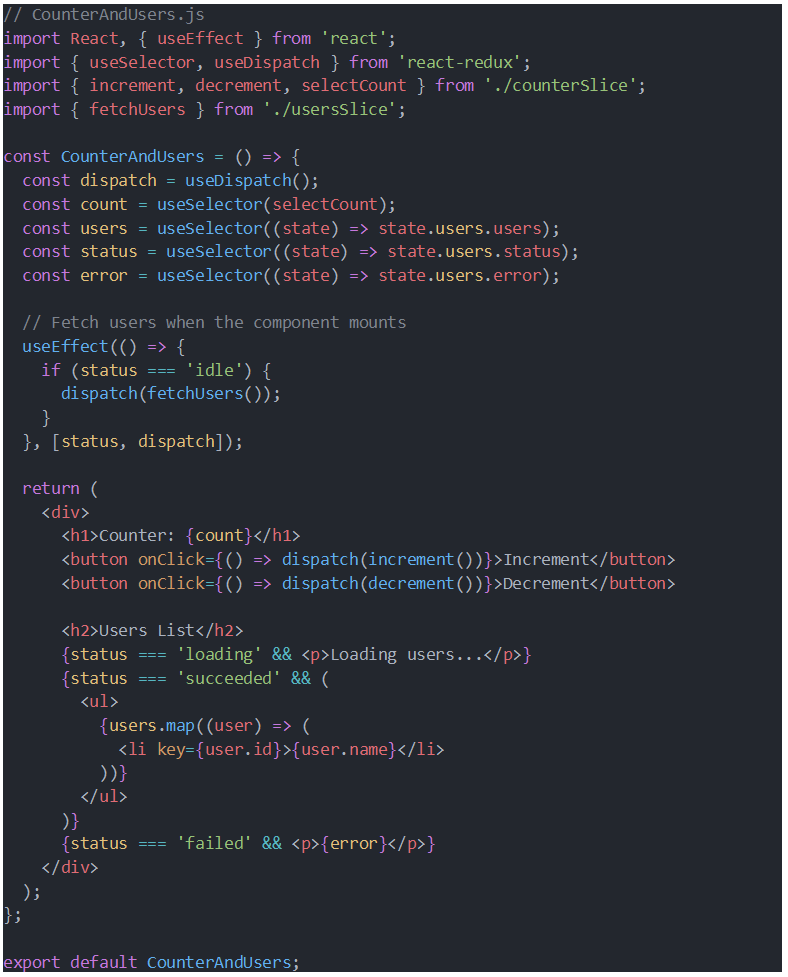
When building React components with Redux Toolkit, useSelector
and useDispatch
are the go-to hooks for accessing and updating the store. They simplify the whole process, making state management far easier to integrate directly into functional components.
useSelector
: Subscribing to the Redux State
The useSelector
hook allows a component to select and subscribe to specific parts of the Redux state. Say you have a counter
slice in your store, useSelector
can pull out the count
value from that slice. This way, components only re-render when the specific part of the state they’re subscribed to changes. No more relying on prop drilling or complex state-passing, useSelector
keeps it direct and focused.
useDispatch
: Dispatching Actions to Change State
On the other hand, useDispatch
enables a component to send actions to the Redux store. It’s how we trigger updates to the state—such as incrementing or decrementing the count in a counter component. By calling useDispatch
and dispatching actions like increment()
or decrement()
, the component directly communicates with Redux to update the state as needed. Together, useSelector
and useDispatch
eliminate the boilerplate that used to come with Redux. They make it possible to both access and modify state without extra setup or complicated connectors, keeping the code clean and focused on logic.
Conclusion
Building an LLM-powered application to chat with documents offers a revolutionary way to interact with and extract information from large volumes of text, particularly within PDF files. By leveraging tools like Langchain, FAISS, and advanced language models from OpenAI or HuggingFace, this solution provides a powerful, user-friendly interface for querying document content.
This approach not only streamlines information retrieval but also enhances the accuracy and efficiency of data extraction processes. Whether in business, research, or other fields, the ability to quickly access and converse with document content gives users a significant edge, allowing them to make informed decisions faster. As AI-driven technologies continue to evolve, applications like this will become indispensable tools for navigating the ever-growing sea of digital information.