In modern web development, ensuring a seamless user experience is crucial. As React applications grow in complexity, manual testing becomes inefficient and error prone. This is where automated UI testing comes in, helping developers catch bugs early and maintain application stability.
Cypress has emerged as a popular choice for end-to-end (E2E) testing, offering a fast, reliable, and developer-friendly way to test React applications. Unlike traditional testing frameworks, Cypress runs directly in the browser, providing real-time feedback and powerful debugging tools.
In this article, we will explore how to set up Cypress for a React project, write effective UI tests, and integrate them into a CI/CD pipeline for continuous quality assurance. Whether you are new to Cypress or looking to improve your test coverage, this guide will equip you with the knowledge to build robust and maintainable UI tests.
Types of testing in React.
- Unit Testing
- Focuses on individual components or functions.
- Ensure components render correctly with different props and states.
- Example tools: Jest, React Testing Library
- Integration Testing
- Tests multiple components working together.
- Checks state management, API calls, and component interactions.
- Example tools: React Testing Library, Cypress, Jest with Mocks
- End-to-End (E2E) Testing
- Tests the full application workflow from a user’s perspective.
- Mimics real-world interactions like form submissions, navigation, and authentication.
- Example tools: Cypress, Playwright, Selenium
- Snapshot Testing
- Captures component output and compares it against a saved snapshot.
- Ensures UI consistency after changes.
- Example tool: Jest
- Performance Testing
- Checks for rendering speed and performance bottlenecks.
- Tools: Lighthouse, React Profiler, Jest Performance Testing
Overview of Cypress and How It Differs from Other Testing Frameworks
Unlike traditional testing tools that run outside the browser, Cypress operates directly within the browser environment, offering real-time feedback, faster execution, and easier debugging.
It is widely used for UI testing in React applications due to its,
- Ease of Setup – No need for complex configurations.
- Fast Execution – Tests run in real-time with automatic reloading.
- Powerful Debugging – Built-in developer tools and time-travel debugging.
- Automatic Waiting – No need for manual wait statements, Cypress intelligently waits for elements to be ready.
How Cypress Differs from Other Testing Frameworks
Feature | Cypress | Selenium | Playwright | Jest + React Testing Library |
Execution Environment | Runs directly in the browser | Uses a WebDriver to interact with browsers | Runs in multiple browsers via automation | Runs in a simulated DOM (JSDOM) |
Ease of Setup | Simple, no extra drivers needed | Requires WebDriver setup | Requires additional setup but supports multiple browsers | Lightweight, but limited to unit tests |
Speed | Fast execution, no network latency | Slower due to WebDriver interactions | Fast and supports parallel testing | Fast, but doesn’t support full UI testing |
Debugging | Time-travel debugging, live reloading | Difficult debugging through logs | Good debugging tools | Debugging via console.logs and snapshots |
Testing Scope | E2E, component, and integration testing | Primarily E2E testing | E2E, API, and component testing | Unit and component testing |
Key Advantages of Cypress
- Developer-Friendly – Cypress provides real-time execution, meaning tests run as you write them.
- Time-Travel Debugging – View snapshots of each test step to analyze what happened.
- Flake-Resistant Tests – Automatically retries failed requests and handles network conditions.
- Built-in Assertions – Comes with powerful built-in assertions for UI interactions.
Cypress is a great choice for React applications where UI consistency and interactivity need thorough testing. While Selenium and Playwright offer multi-browser support, Cypress stands out with its simplicity, speed, and developer-focused debugging capabilities.
Setting Up Cypress in a React Project
Cypress is easy to install and configure in a React project, making it a smart choice for automated UI testing. Here’s a step-by-step guide to getting started:
1. Install Cypress
First, navigate to your React project folder and install Cypress via npm or yarn:

This installs Cypress as a development dependency, keeping it separates from your production build.
2. Open Cypress for the First Time
Once installed, you can launch Cypress with:

This command will:
- Create a default Cypress folder structure (
cypress/
) in your project. - Open the Cypress Test Runner, where you can run and manage your tests.
- Generate example test files inside
cypress/e2e/
to help you get started.
3. Cypress Folder Structure
After running Cypress, your project will have a folder structure like this:
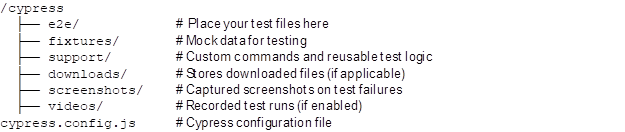
4. Configure Cypress for Your Project
You can customize Cypress settings in cypress.config.js
(or cypress.config.ts
for TypeScript projects). Here’s a basic example:
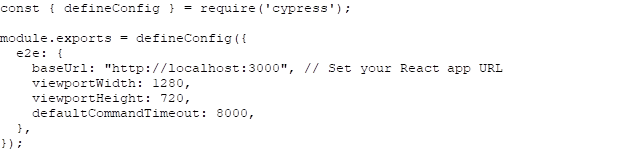
If your React app runs on a different port (e.g., Vite defaults to http://localhost:5173
), update the baseUrl
accordingly.
5. Writing Your First Cypress Test
Create a new test file inside cypress/e2e/
, for example, home.cy.js
:

This test:
- Visits the home page (
/
). - Checks if the text “Welcome to React” is visible.
We can run the test with:

Introduction to Form Testing in Cypress
- Forms are crucial in web applications (user sign-ups, logins, contact forms, etc.).
- Testing ensures correct validations, submissions, and API interactions.
- Cypress makes form testing easy by simulating user interactions.
1. Writing a Basic Cypress Test for a Form
Example: Testing a Login Form
Assume we have a simple React form with two inputs and a submit button:

Cypress Test:
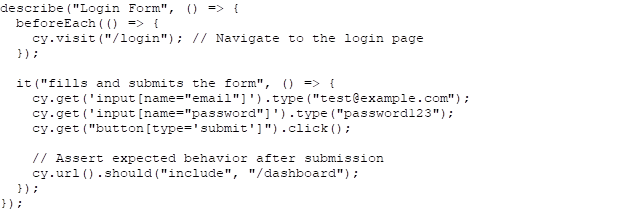
cy.get()
Selects the elements using CSS selectors or data attributes.type()
to simulate user input.click()
to submit.should()
to assert the expected result (redirect after successful login).
2. Handling Form Validations
Example: Ensuring Error Messages Appear

- Ensures validation works properly.
- Asserts error messages appear when expected.
3. Testing API Calls with cy.intercept()
- Mocking API responses prevents reliance on actual backend behavior.
cy.intercept()
allows us to control API responses in tests.- It gives the ability to simulate different API responses (success, error, delays).
Example: Mocking a Login API Call
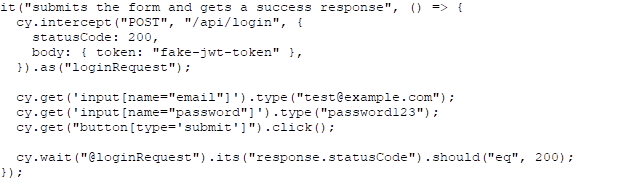
cy.intercept()
mocks the API response.as("loginRequest")
gives the call an alias for tracking.cy.wait("@loginRequest")
ensures Cypress waits for the request.- Assertions validate the response status.
4. Testing Error Responses
Example: Handling 401 Unauthorized Response
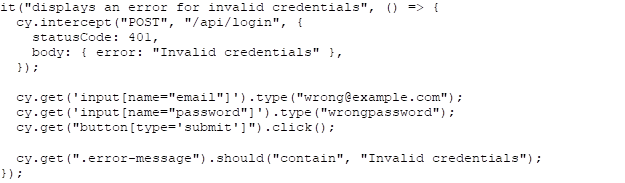
This ensures that the UI handles authentication failures properly.
Component Testing with Cypress in React
Cypress is widely known for end-to-end (E2E) testing, but it also offers component testing, which allows you to test React components in isolation. This ensures your components work as expected before integrating them into full-page UI tests.
1. Why Use Cypress for Component Testing?
Cypress can also be an effective tool for component testing due to its robust capabilities, ease of use, and features tailored for testing the front-end. Using Cypress for component testing in React applications offers several advantages.
- Runs components in a real browser environment.
- Supports interactive debugging.
- Provides fast feedback compared to full E2E tests.
- Works well with popular React libraries (e.g., Material UI, Tailwind, Redux, etc.).
2. Setting Up Cypress for Component Testing
Step 1: Install Cypress|
If you haven’t installed Cypress yet, add it to your project.
Step 2: Install Cypress Component Testing Dependencies

Step 3: Configure Cypress for Component Testing
Modify your cypress.config.js
or cypress.config.ts file accordingly.
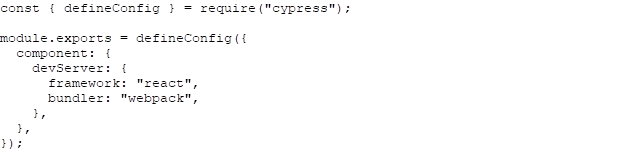
3. Writing Your First Cypress Component Test
Let’s test a simple Button component.
Example Component: Button.jsx

Component Test: Button.cy.js
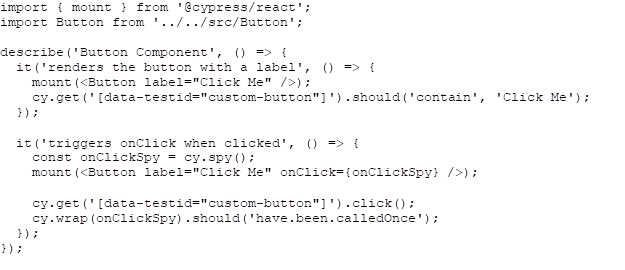
- mount(<Button label=”Click Me” />) mounts the Button component in isolation.
- cy.get(‘[data-testid=”custom-button”]’) targets the button by its data-testid attribute.
- should(‘contain’, ‘Click Me’) asserts that the button contains the label “Click Me”.
- const onClickSpy = cy.spy() creates a spy function that will track how many times it’s called.
- mount(<Button label=”Click Me” onClick={onClickSpy} />) mounts the Button component with the onClick handler passed as the spy.
- cy.get(‘[data-testid=”custom-button”]’).click() simulates a click event on the button.
- cy.wrap(onClickSpy).should(‘have.been.calledOnce’) asserts that the spy function was called exactly once.
4. Running Cypress Component Tests
To start Cypress in component testing mode, run:
Then, select your test file (Button.cy.js
) from the Cypress UI, and the tests will run in real-time.
5. Best Practices for Cypress Component Testing
- Use data-testid for Selecting Elements – This ensures stable element selection and avoids breaking tests due to CSS changes.
- Use spy() and cy.stub() for Mocking Functions – Helps verify event handlers like button clicks without modifying the component.
- Test Component Props and States – Ensure the component correctly handles different props and updates states properly.
- Use beforeEach() to Reduce Redundant Code

6. Advanced Component Testing Features
- Mocking API Calls in Components
If your component fetches data, use cy.intercept()
to mock responses.

- Testing Components with Redux or Context API
Use mount()
with a provider wrapper.
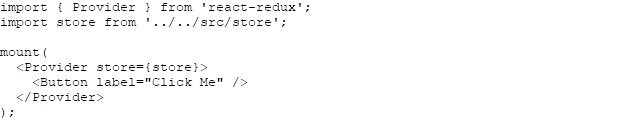
Best Practices for Writing Cypress Tests in React
Cypress is a powerful tool for testing React applications, but to ensure your tests are reliable, maintainable, and performant, it’s suitable to follow these best practices:
1. Use data-testid
for Stable Element Selection
Relying on CSS classes or IDs for element selection can make tests fragile due to frequent UI changes. Instead, use data-testid(data-cy)
attributes for more stable selectors.
Good Practice:

Bad Practice:

2. Avoid Using cy.wait()
for Timing Issues
Hardcoded waits (cy.wait(5000)
) slow down tests and make them unreliable. Instead, use assertions and built-in automatic waiting in Cypress.
Good Practice:

Bad Practice:

3. Keep Tests Independent and Isolated
Each test should set up its own state and not depend on previous tests.
Good Practice:
- Use
beforeEach() function
to reset the application state before each test.

- Use
cy.intercept()
to stub API responses.
4. Use Fixtures and Mock API Calls
Avoid making real API requests in tests. Instead, mock responses to ensure consistency.
Mock API Response:

Fixtures (cypress/fixtures/user.json
)

5. Test Only What Matters (Avoid Over-Testing UI Details)
Don’t test third-party libraries or React internals. Focus on user interactions and expected behavior.
Test user behavior:

Avoid testing UI structure:

6. Use cy.wrap()
for Custom Assertions
When dealing with asynchronous behavior, use cy.wrap()
to assert custom logic.

7. Run Tests in Headless Mode for CI/CD
For faster execution in CI/CD, use headless mode:

Conclusion
Automating UI tests in React with Cypress provides a robust and efficient way to ensure application reliability. By leveraging Cypress’ powerful testing features, developers can write clear, maintainable, and stable tests while avoiding common pitfalls like flaky tests and unreliable network calls.
Key Takeaways
- Set up Cypress properly in a React project to streamline UI testing.
- Structure test cases for readability using the Arrange-Act-Assert pattern.
- Use custom commands to avoid repetitive test logic and improve maintainability.
- Handle network requests efficiently with
cy.intercept()
to mock API responses and test various scenarios. - Run tests in headless mode to integrate with CI/CD pipelines and automate testing workflows.
- Improve test reliability by avoiding hardcoded waits, handling slow responses, and testing error handling.
By implementing these best practices, teams can build a robust test suite that ensures smooth user experience and minimizes regressions. Cypress makes UI testing accessible, fast, and scalable—empowering developers to ship high-quality applications with confidence.