Introduction
Playwright and its advantages for API automation
In today’s digital age, automation is the key to efficiency. Playwright, a powerful automation tool, simplifies the process of testing and interacting not only with web applications but also with APIs. In this guide, we’ll explore how to automate APIs using Playwright, even if you’re new to the tool.
Before we dive into the practical aspects, let’s briefly understand what Playwright is and why it’s a great choice for API testing.
- Multi-Browser Support:
Test your APIs across multiple browsers, ensuring compatibility and consistency. - Easy Setup:
Playwright’s simple installation process and clear documentation make it accessible to beginners. - Powerful Assertions:
Easily verify API responses and assert specific conditions with Playwright’s built-in assertion methods. - Detailed Reporting:
Get comprehensive reports on test results, including screenshots, to quickly identify and address issues.
Page Object Model (POM) approach for structuring test automation code in API automation.
In API automation using Playwright, the Page Object Model (POM) is a design pattern that helps organize and manage the interactions with API endpoints in a structured and reusable way. While POM is traditionally associated with UI testing, it can also be applied effectively to API testing scenarios.
What is Page Object Model (POM)?
The Page Object Model (POM) is a design pattern used to represent web pages or components in UI automation testing. It encapsulates the elements of a page and the actions that can be performed on those elements into separate classes or modules, known as Page Objects.
Adapting POM for API Testing with Playwright
In the context of API testing with Playwright, we can adapt the principles of POM to represent API endpoints and the actions performed on those endpoints. Instead of UI elements, Page Objects in API automation represent the API endpoints and the methods to interact with them.
Benefits of Using POM in API Automation with Playwright
Modularity:
Page Objects provide a modular approach to organizing API endpoints, making it easier to manage and maintain the test code.
Readability:
By encapsulating API interactions within Page Objects, test scripts become more readable and understandable, even for those new to automation.
Reusability:
Page Objects can be reused across multiple test scripts, promoting code reusability, and reducing duplication of code.
Maintenance:
Updates or changes to API endpoints can be managed within the corresponding Page Objects, minimizing the impact on test scripts.
Prerequisites
Basic Understanding of JavaScript:
Since Playwright scripts are written in JavaScript in the below steps, having a fundamental understanding of JavaScript syntax, variables, data types, functions, and control structures is crucial.
Knowledge of Asynchronous Programming:
JavaScript is inherently asynchronous, so understanding concepts such as promises, async/await, and handling asynchronous operations is important for writing efficient Playwright scripts.
Familiarity with RESTful APIs:
Knowledge on RESTful APIs, including how they work, common HTTP methods (GET, POST, PUT, DELETE), request headers, query parameters, request/response formats (JSON, XML), and status codes.
Setup Node.js and npm:
Install Node.js, which includes npm (Node Package Manager), on your development environment. Node.js allows you to run JavaScript outside the browser, which is necessary for running Playwright scripts.
Text Editor or IDE:
Choose a text editor or integrated development environment (IDE) for writing your JavaScript code. The most popular option includes Visual Studio Code.
Once you’ve done these things, you’ll have what you need to start learning how to automate APIs using Playwright and JavaScript, even if you’re just starting. Make sure to practice often and try out different situations. This will help you get better at testing APIs with Playwright. Now, let’s jump into the practical aspect of using Playwright for API testing.
Explore the practical aspects
Step 1: Installation
First, you need to create your project folder in the system. Then open your VS code editor and open the folder that you have already created, using the editor.
Start by installing the latest version of Playwright using npm (Node Package Manager). Open your terminal or command prompt and run the following command.
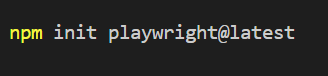
This command will create a “package.json” file in your project folder with default settings. Also “playwright.config.js” file which contains the required playwright configurations will be created. Apart from that, the tests folder also getting created with some sample and demo tests.
Step 2: Writing Your First API Test
Begin your journey by opening your favourite code editor. Start by defining Page Objects to represent the API endpoints or resources you’ll be testing. Each Page Object should encapsulate the URL, request parameters, headers, and methods for interacting with the API.
As we embark on this adventure, let’s walk through the creation of Page Objects for API requests and responses using a POST API as an example.
- Navigate to the project folder in your code editor or file explorer.
- Create a new folder and give it a meaningful name that reflects its purpose, such as “apiPageObjects”.
- Within the newly created folder, organize your page objects into separate JavaScript files. Page objects are essentially modules that encapsulate the functionality and data related to specific API endpoints or resources.
- Inside the folder, create two JavaScript files for holding information related to the API request and response.
- Choose descriptive names for your JavaScript files that indicate their purpose or the API endpoint/resource they represent, such as “apiRequest.js” and “apiResponse.js”.
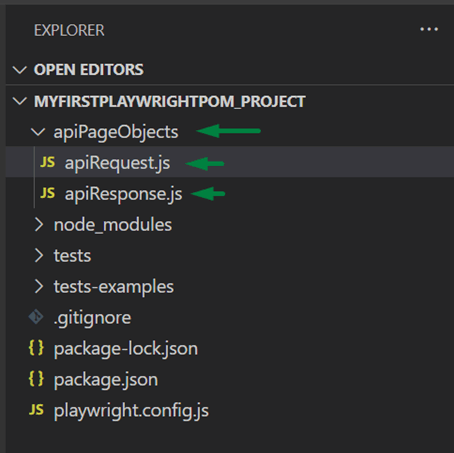
Now let’s explore how to write the code in each .js file. Remember to export the modules as they are essential for executing the test under the test file.
This is what your POST API request looks like:
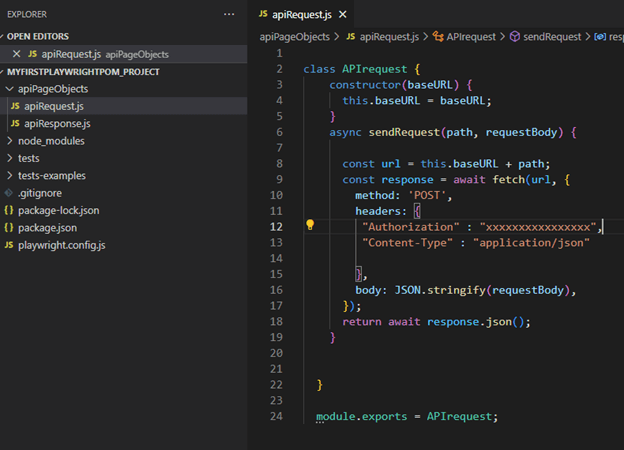
This is what your POST API response looks like:
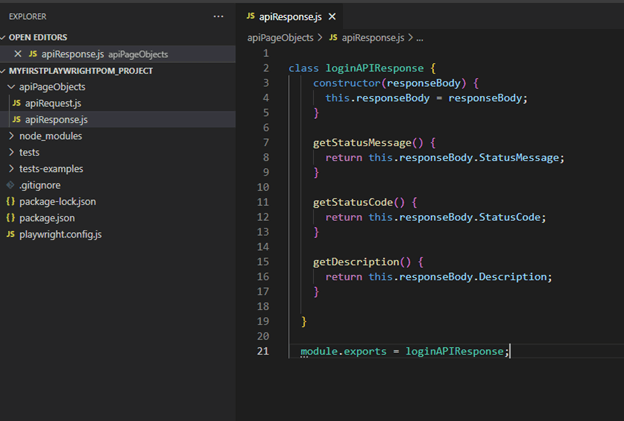
Now, let’s jump into the most important step of test creation. When creating tests, import the relevant page objects for the API request and response. Utilize the functions provided by these page objects to interact with the API and extract necessary data from the response.
Write test cases that define the expected behavior of the API under various conditions. This may include verifying the correctness of the response data, checking for error handling, and validating edge cases.
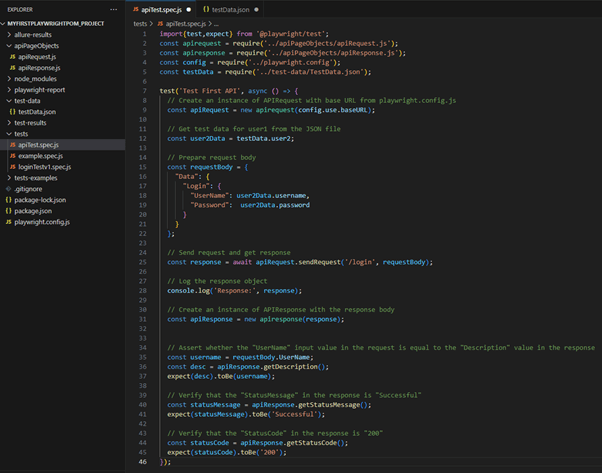
Here’s a brief explanation of the code in the “apiTest.spec.js”
- import the Playwright library at the beginning of the file.
- import apiRequest.js file that was created in the earlier steps. Remember that it is mandatory to export it in the relevant .js file to import in the test spec file.
- import apiResponse.js file that was created in the earlier steps. Remember that it is mandatory to export it in the relevant .js file to import in the test spec file.
- import playwright.config.js which configured the base URL of the API endpoint.
- import data file which contains required input data for API request using the testData.json file.
Note:
Data-driven testing allows you to execute the same test scenario with multiple sets of test data. This approach enhances test coverage and helps identify edge cases. Instead of writing separate test cases for each data scenario, you use a single test case and feed it different inputs. This approach helps you validate how your application behaves with different data inputs and ensures robustness across various conditions.
Line #34-45 – You will be able to assert your status code as well as response body as you wish, using Playwright’s built-in assertion methods.
Step 3: Running Your Test
Playwright’s flexible test execution options cater to various testing needs, from simple single test runs to complex multi-browser setups, while offering enhanced debugging capabilities.
Playwright offers versatile options for test execution:
Test Selection: You can run a single test, a group of tests, or all tests in your suite.
Command to run all the tests in the test folder:
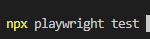
Command to run a single test:

Browser Configuration: Tests can be executed on a single browser or multiple browsers using the –project flag, allowing for cross-browser testing.
Parallel Execution: Tests are executed in parallel by default, enhancing efficiency.
Headless Mode: Tests run in headless mode by default, meaning no browser window is displayed during execution. Results are shown in the terminal.
Headed Mode: You can opt to run tests in headed mode using the –headed CLI argument, which displays the browser window during execution.
UI Mode: Alternatively, you can run tests in UI mode with the –ui flag, providing a graphical user interface for test execution.
Additional Features: Playwright offers features like watch mode and time travel debugging, providing a comprehensive trace of test execution for debugging and analysis.
Step 4: Analysing Test Results
Reporters help in reporting the test execution results in a readable and actionable format. They enhance visibility into test outcomes, making it easier to understand the status of the tests and identify any failures or errors. Playwright supports various types of reporters, including built-in reporters and third-party plugins. Some common types include:
Console Reporter: Displays test results in the terminal/console in a structured format.
JUnit/XML Reporter: Generates XML output compatible with JUnit, which can be consumed by CI/CD systems and other reporting tools.
HTML Reporter: Creates HTML reports with detailed test execution information, including passed, failed, and skipped tests, along with any error messages and stack traces.
JSON Reporter: Outputs test results in JSON format, which can be parsed and analysed programmatically or used for custom reporting.
Allure Reporter: Integrates with the Allure framework to generate interactive and visually appealing reports with rich features like test history, attachments, and trends analysis.
Customization: Reporters in Playwright often offer customization options, allowing you to tailor the output format and content according to your requirements. You can also configure settings such as output file paths and additional metadata to include in the reports.
To use a reporter in Playwright, you typically specify it as a command-line argument or configure it in your test runner configuration file.
For example:
To Generate the JUnit report using the terminal

To generate the .html report using the terminal

View the .html report.

Here is a sample .html report after executing the “npx playwright show-report” command.
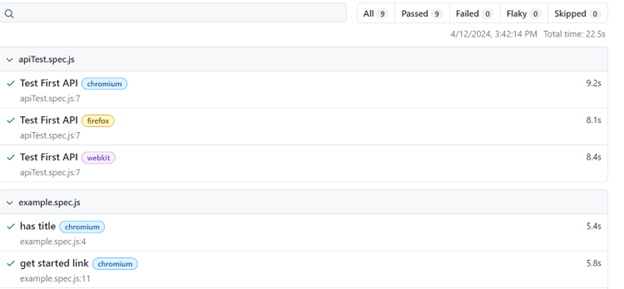
Overall, reporters in Playwright play a crucial role in providing insights into test execution results, facilitating collaboration among team members, and ensuring the reliability of your test automation efforts.
Best Practices for Playwright API Testing:
Clear and Modular Code:
Write clear, modular, and reusable code by following best practices such as proper naming conventions, encapsulation, and separation of concerns. This ensures that your test scripts are easy to understand, maintain, and scale.
Page Object Model (POM):
Implement the Page Object Model to encapsulate API endpoints, request parameters, and response handling logic. This design pattern promotes code reusability and maintainability by separating test logic from implementation details.
Data-Driven Testing:
Use data-driven testing techniques to run tests with multiple sets of input data. This helps validate different scenarios and edge cases, enhancing test coverage and robustness.
Assertions and Error Handling:
Use meaningful assertions to validate API responses against expected outcomes. Implement robust error handling mechanisms to gracefully handle exceptions and failures, providing informative error messages for debugging.
Environment Configuration:
Manage environment-specific configurations, such as base URLs and authentication tokens, using configuration files or environment variables. This allows you to easily switch between different environments (e.g., development, staging, production) without modifying test code.
Parameterization:
Parameterize your test scripts to make them more flexible and configurable. Use variables or configuration files to store dynamic values like request payloads, headers, and authentication tokens.
Test Isolation and Cleanup:
Ensure test isolation by setting up and tearing down test fixtures (e.g., test data, API state) before and after each test execution. This prevents test dependencies and side effects, ensuring reliable and reproducible test results.
Continuous Integration (CI):
Integrate API tests into your CI/CD pipeline to automate test execution and ensure early detection of regressions. Use CI tools like Jenkins or GitHub Actions to run tests automatically on every code change.
Logging and Reporting:
Implement logging mechanisms to capture relevant information during test execution, such as request/response details and execution timestamps. Integrate with reporting tools or frameworks (e.g., Allure, Jest) to generate comprehensive test reports with actionable insights.
Documentation and Collaboration:
Document your test scripts, including test scenarios, preconditions, and expected outcomes, to facilitate collaboration and knowledge sharing among team members. Use version control systems (e.g., Git) to manage test code and track changes over time.
References
Playwright official documentation – https://playwright.dev/
Conclusion:
Playwright provides a powerful and flexible platform for API testing, allowing developers and testers to automate the validation of their backend services with ease. By leveraging the rich features of Playwright, such as the Page Object Model, data-driven testing, and robust error handling, teams can create reliable and maintainable API test suites.
With Playwright, you can execute tests in parallel, run them headless or in UI mode, and integrate seamlessly with CI/CD pipelines for continuous testing. The ability to write tests in JavaScript, TypeScript, or Python provides flexibility and caters to diverse skill sets within the team.
Furthermore, by following best practices such as clear and modular code, proper error handling, and integration with reporting tools, teams can ensure the quality and reliability of their API test automation efforts.
Incorporating Playwright into your testing workflow empowers teams to streamline their testing processes, improve test coverage, and accelerate the delivery of high-quality software products.
Embrace the power of Playwright for API testing and elevate the quality of your software applications today!